Viewing Progress
Progress Bar
Passing the verbose=True argument to the Contour.roots method or find_roots function will cause a progress bar to be printed:
from cxroots import Circle
C = Circle(0, 3)
f = lambda z: (z + 1.2) ** 3 * (z - 2.5) ** 2 * (z + 1j)
C.roots(f, verbose=True)
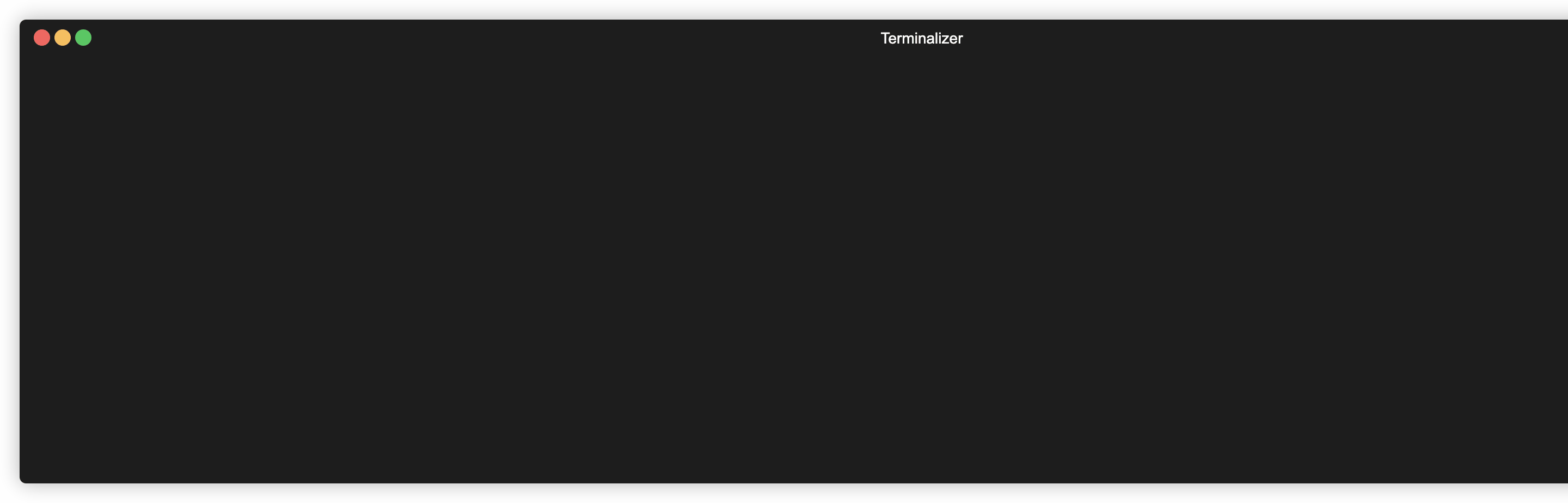
Logging
More detailed runtime information is available using Python’s standard logging module, for example:
import logging
from numpy import exp, cos, sin
from cxroots import Circle
C = Circle(0, 3)
f = lambda z: (exp(-z) * sin(z / 2) - 1.2 * cos(z)) * (z + 1.2) * (z - 2.5) ** 2
# use logging.DEBUG to show diagnostic information
logging.basicConfig(level=logging.INFO)
C.roots(f)
INFO:cxroots.root_counting:Counted 6 roots (including multiplicities) within Circle: center=0.000+0.000i, radius=3.000
INFO:cxroots.root_finding:Subdividing Circle: center=0.000+0.000i, radius=3.000
INFO:cxroots.root_counting:Counted 6 roots (including multiplicities) within Annulus: center=0.000+0.000i, inner radius=0.900, outer radius=3.000
INFO:cxroots.root_counting:Counted 0 roots (including multiplicities) within Circle: center=0.000+0.000i, radius=0.900
INFO:cxroots.root_finding:Subdividing Annulus: center=0.000+0.000i, inner radius=0.900, outer radius=3.000
INFO:cxroots.root_counting:Counted 3 roots (including multiplicities) within Annulus sector: center=0.000+0.000i, r0=0.900, r1=3.000, phi0=1.885, phi1=5.027
INFO:cxroots.root_counting:Counted 3 roots (including multiplicities) within Annulus sector: center=0.000+0.000i, r0=0.900, r1=3.000, phi0=5.027, phi1=8.168
INFO:cxroots.root_approximation:Approximating the 3 roots in: Annulus sector: center=0.000+0.000i, r0=0.900, r1=3.000, phi0=5.027, phi1=8.168
INFO:cxroots.root_finding:Subdividing Annulus sector: center=0.000+0.000i, r0=0.900, r1=3.000, phi0=5.027, phi1=8.168
INFO:cxroots.root_counting:Counted 1 roots (including multiplicities) within Annulus sector: center=0.000+0.000i, r0=0.900, r1=1.530, phi0=5.027, phi1=8.168
INFO:cxroots.root_counting:Counted 2 roots (including multiplicities) within Annulus sector: center=0.000+0.000i, r0=1.530, r1=3.000, phi0=5.027, phi1=8.168
INFO:cxroots.root_approximation:Approximating the 2 roots in: Annulus sector: center=0.000+0.000i, r0=1.530, r1=3.000, phi0=5.027, phi1=8.168
INFO:cxroots.root_finding:Recorded root (2.5+3.0703047868410416e-163j) with multiplicity 2
INFO:cxroots.root_approximation:Approximating the 1 roots in: Annulus sector: center=0.000+0.000i, r0=0.900, r1=1.530, phi0=5.027, phi1=8.168
INFO:cxroots.root_finding:Recorded root (1.440251130166703-4.503215788022396e-26j) with multiplicity 1
INFO:cxroots.root_approximation:Approximating the 3 roots in: Annulus sector: center=0.000+0.000i, r0=0.900, r1=3.000, phi0=1.885, phi1=5.027
INFO:cxroots.root_finding:Subdividing Annulus sector: center=0.000+0.000i, r0=0.900, r1=3.000, phi0=1.885, phi1=5.027
INFO:cxroots.root_counting:Counted 1 roots (including multiplicities) within Annulus sector: center=0.000+0.000i, r0=0.900, r1=1.530, phi0=1.885, phi1=5.027
INFO:cxroots.root_counting:Counted 2 roots (including multiplicities) within Annulus sector: center=0.000+0.000i, r0=1.530, r1=3.000, phi0=1.885, phi1=5.027
INFO:cxroots.root_approximation:Approximating the 2 roots in: Annulus sector: center=0.000+0.000i, r0=1.530, r1=3.000, phi0=1.885, phi1=5.027
INFO:cxroots.root_finding:Subdividing Annulus sector: center=0.000+0.000i, r0=1.530, r1=3.000, phi0=1.885, phi1=5.027
INFO:cxroots.root_counting:Counted 1 roots (including multiplicities) within Annulus sector: center=0.000+0.000i, r0=1.530, r1=3.000, phi0=1.885, phi1=2.827
INFO:cxroots.root_counting:Counted 1 roots (including multiplicities) within Annulus sector: center=0.000+0.000i, r0=1.530, r1=3.000, phi0=2.827, phi1=5.027
INFO:cxroots.root_approximation:Approximating the 1 roots in: Annulus sector: center=0.000+0.000i, r0=1.530, r1=3.000, phi0=2.827, phi1=5.027
INFO:cxroots.root_finding:Recorded root (-0.9746510351110598-1.3810477682471562j) with multiplicity 1
INFO:cxroots.root_approximation:Approximating the 1 roots in: Annulus sector: center=0.000+0.000i, r0=1.530, r1=3.000, phi0=1.885, phi1=2.827
INFO:cxroots.root_finding:Recorded root (-0.9746510351110598+1.3810477682471562j) with multiplicity 1
INFO:cxroots.root_approximation:Approximating the 1 roots in: Annulus sector: center=0.000+0.000i, r0=0.900, r1=1.530, phi0=1.885, phi1=5.027
INFO:cxroots.root_finding:Recorded root (-1.2+0j) with multiplicity 1
INFO:cxroots.root_finding:Completed rootfinding with 92321 evaluations of the given analytic function at 92321 points
Multiplicity | Root
------------------------------------------------
1 | -1.200000000000 +0.000000000000i
1 | -0.974651035111 -1.381047768247i
1 | -0.974651035111 +1.381047768247i
1 | 1.440251130167 -0.000000000000i
2 | 2.500000000000 +0.000000000000i
Logging with Progress Bar
To get the logs to neatly stream above the progress bar the logging config needs to be passed the RichHandler like so:
import logging
from rich.logging import RichHandler
from numpy import exp, cos, sin
from cxroots import Circle
C = Circle(0, 3)
f = lambda z: (exp(-z) * sin(z / 2) - 1.2 * cos(z)) * (z + 1.2) * (z - 2.5) ** 2
logging.basicConfig(level=logging.INFO, handlers=[RichHandler()])
C.roots(f, verbose=True)
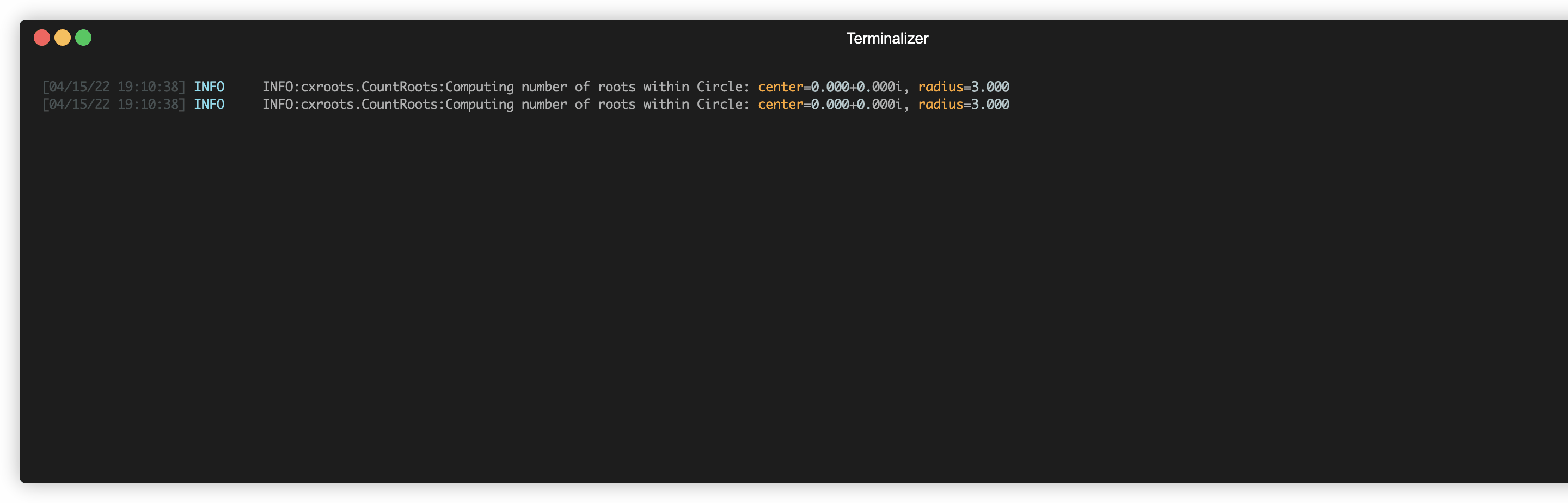